javascript ES6
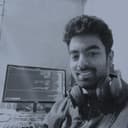
Rukon Uddin
08/25/2024
ES6 refers to version 6 of the ECMA script programming language. … is a major extension of the JavaScript language, and adds many more features aimed at simplifying large-scale software development. ECMAScript, or ES6, was published in June 2015. It was later renamed ECMAScript 2015.
Variable:
1. Here are 3 ways to declare a JavaScript variable:
- Var
- Let
- Const
A variable is a container for storing data
Below is how to declare a variable:
Example:
- var: var keyword is a global scope variable declared.
var year=20; console.log(name,year); console.log(name,year);
- var: Let: The keyword let declare a block scope variable. It is confined within the scope. The variable cannot be re-declared with let, but its value can be changed.
Example:1
let name = "Rukon"; let name= "Delwar"; // SyntaxError: 'x' has already been declared
Example:2
{ let number = 2; } console.log(number) // x can NOT be used here
Example:3
Let number=100; number=200; console.log(number); // number is 200
- Const: The const keyword declares a JavaScript variable with a constant value. Constants are like let variables, values cannot be changed. With const Declared variable blocks have scope.
Example: 1
var a = 10; { const a = 2; } console.log(a) // output= 10
Example: 2
const number = 1000; number = 122; // This will give an error number = number + 10; // This will also give an error
Default parameters:
- The default function allows the parameters named parameters to start with the default value if there is no value or unspecified pass.
Example:
function multiply(a, b = 1) { return a * b; } console.log(multiply(5, 2)); // expected output: 10 console.log(multiply(5)); // expected output: 5
Template string:
- Template use back-tick () instead of quotes ("") to define a string.
Example: 1
let template = `Template string`;
In Template Literal, both single and double quotes can be used inside a string.
Example: 2
let text = `I am "rukon"`;
- The template allows literally multi line strings
Example: 3
let text = `The template allows literally multiline strings`;
- Template literals provide an easy way to expand variable expressions into strings. This method is called string interpolation.
Example: 4
let name= "Rukon"; let year= 20; let person= `my name is ${name}.I am ${year}!years old`; console.log(person)
Spread Operator:
- The JavaScript spread operator (…) can copy all data from an existing array or object to another array or object.
Example:
const numbersOne = [1, 2, 3]; const numbersTwo = [4, 5, 6]; const numbersCombined = […numbersOne, …numbersTwo]; console.log(numbersCombined) // outpot 1, 2, 3,4, 5, 6
Arrow Functions:
- The arrow function allows us to write the short function syntax:
We used to write functions like this:
Example:1
Function before() { return "Before Function!"; } console.log(before)
Arrow Function:
Example:2
const arrow = () => { return "Arrow Function!"; } console.log(arrow())
- If the function does not need to be a parameter and returns a single value, then the direct function can be written without a cursor. Then the function becomes smaller.
Example:3
const arrow = () => "Arrow Function";